Add live instructions using a webhook
To add live instructions:
- Fetch the required user data from your system (example in Next.js).
- Create a webhook in Moveo.
- Add a webhook action when the AI Agent initiates, typically in the Greetings dialog.
- Code
- Create webhook
- Dialog
api/live-instructions.ts
import { NextApiRequest, NextApiResponse } from "next";
import * as API from "./api";
const handler = async (req: NextApiRequest, res: NextApiResponse) => {
// Retrieve user ID from the conversation context
const user_id = req.body.context.user_id;
// Fetch user details from your API
const user_info = await API.getUserInfo(user_id);
// Extract required variables from the API response
const { name, transactions, atm } = user_info;
const latest_transaction = transactions[0];
// Format live instructions
const live_instructions = `1. User Name: ${name}
2. Recent Activity: ${name} made a ${latest_transaction.type} of ${latest_transaction.amount} ${latest_transaction.currency}, which is pending and expected to be completed in 2–4 days.
3. The nearest ATM is located at ${atm.address}.`;
return res.json({
context: {
live_instructions,
},
});
};
export default handler;
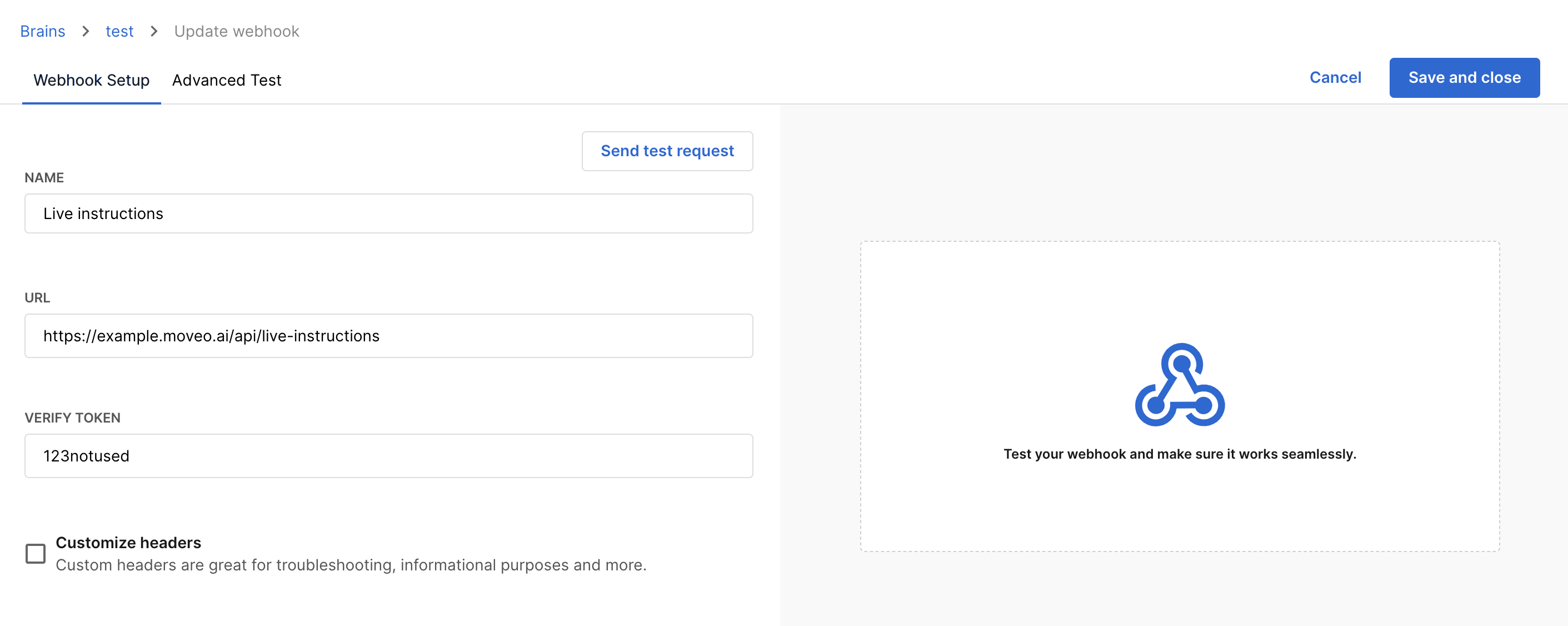
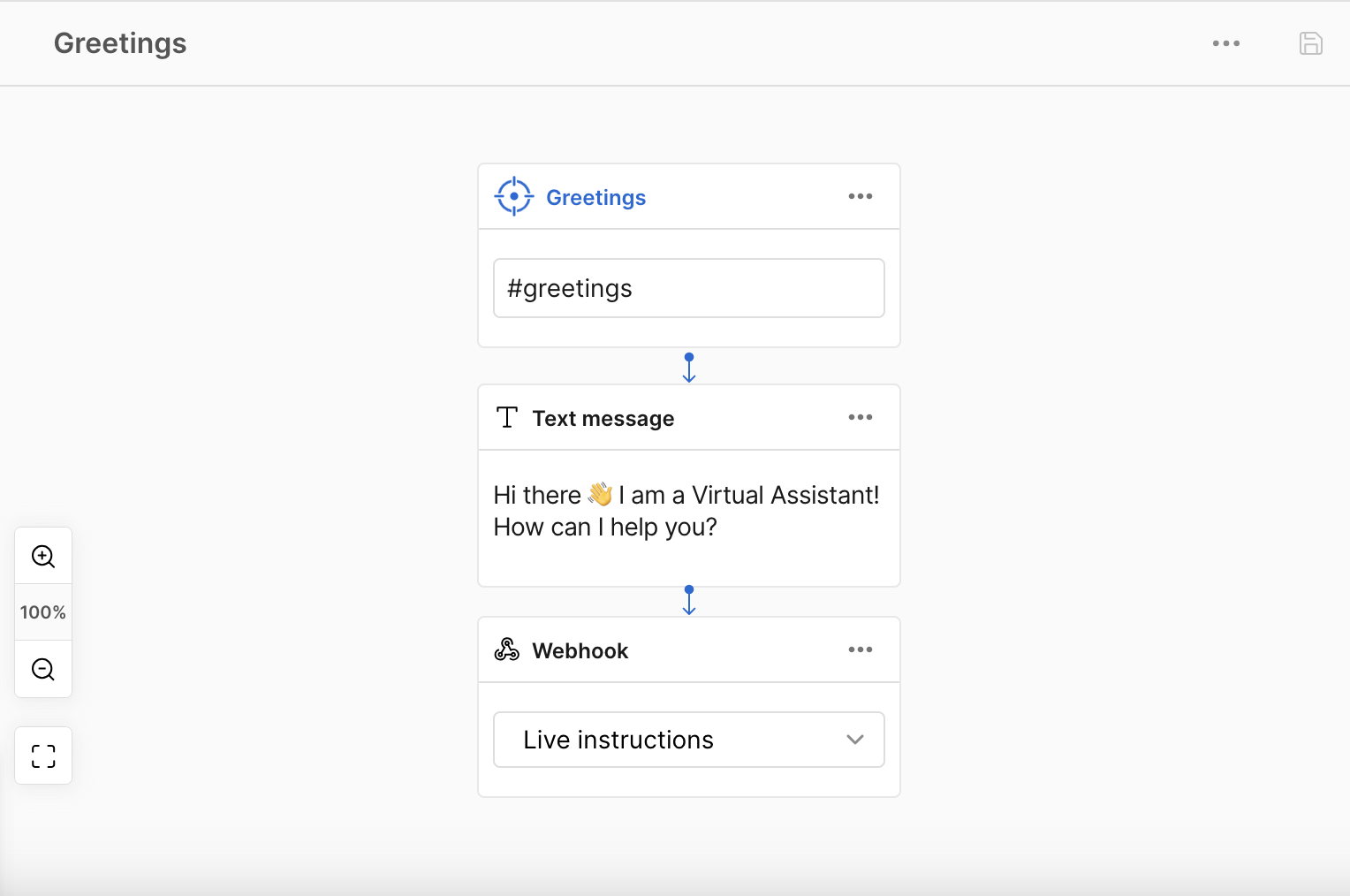
With live instructions, the AI Agent can personalize responses using real-time user data.
tip
Ensure that the information passed to the AI is well-structured and formatted clearly using newline (\n
) and tab (\t
) characters for better readability.